codeheart.js is my easy-to-use framework for making web and mobile games in Javascript. To write networked multiplayer games, you need two additional pieces of technology: a way to run Javascript on the web server and an API for reasonably efficient connection-based communication between the client and server.
The best of breed solutions for these two pieces appear to be Node.js and Socket.IO. Coming to these without any prior experience in the web programming world, it took me a while to figure out how to install and launch these. Once you get them running, they are fairly easy to use for anyone with moderate Javascript experience. Here is some information on how to get to that point.
Node.js is a server-side Javascript interpreter with a built-in library for the kinds of tasks that you'll probably want to perform on a server. You can write a game server in Javascript using it. Socket.IO is a package that extends Node.js servers with the ability to establish fast connections to Javascript clients. It wraps the WebSocket API with some utility methods and fallbacks for older browsers.
Download Node.js from http://nodejs.org/ for your operating system (e.g., Windows, Linux, OS X). On Windows it installs to the Program Files directory (C:\Program Files\nodejs).
The default installation is read-only; you'll have to remove the read only flag (right click on the directory in Windows, use chmod on Unix-based operating systems) in order to install new packages. On windows, I had to manually recurse into the node_modules/npm directory and remove the read-only flag again.
Using your regular shell (Terminal, CMD, bash, etc.), run the "npm" command that comes with Node.js to install new packages. You have to run this as administrator/root. On Windows, create a shortcut to "cmd", and then right-click on the short cut and "run as administrator". You now have an administrator shell. On Unix, just prefix the following command with "sudo" to run as a super user.
In your project directory, execute:
npm install socket.io
at the command line to install the Socket.IO library for this project. Node.js requires you to either install it for each project or install it globally (with the -g flag) and then use a "npm link", which does not work on Windows and is not friendly to revision control systems because it relies on symlinks.
On Windows, I received some errors about an inability to compile the native binaries for Socket.IO, despite having Visual Studio and Python installed as required. On OS X Mountain Lion it worked without error.
To launch your game server, you'll run the Node.js binary (on Windows, node.exe) and pass it the name of the Javascript file that is the server.
A simple server example is not a game server but a web server (yes, a web server, written *in* Javascript.) To try this, put the following code into a file called example.js anywhere on your hard drive:
var http = require('http'); var dns = require('dns'); var os = require('os'); function clientIP(request) { return (request.headers['x-forwarded-for'] || request.connection.remoteAddress); } http.createServer(function (request, response) { console.log('' + clientIP(request) + ' requests ' + request.url); response.writeHead(200, {'Content-Type': 'text/plain'}); response.end('Hello World\n'); }).listen(8124); dns.lookup(os.hostname(), function (err, addr, fam) { console.log('Server running at http://127.0.0.1:8124/ (http://' + addr + ':8124)'); })
Then, at an administrator shell, run:
node example.js
You may need to type a full path in front of node or example.js if you did not store them in the same directory. Open a web browser on that machine and visit:
http://127.0.0.1:8124/
(this is the localhost IP address for your machine, and the port that example.js told the web server to run on). You'll see a document in your web browser that says "Hello World". It should look something like the image above. If it does, then congratulations, you just ran your first server on Node.js! Press Ctrl-C in the command prompt to kill it.
From here, the next step is to read the Node.JS and Socket.IO documentation. They provide lots of useful libraries. The core of what you need for a networked multiplayer game is the same as for a single-player game of course: a lot of Javascript code implementing your world and your UI.
See my next post for more information on launching your first Socket.IO program.
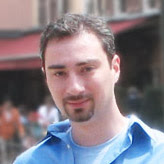